Table of Contents
- Introduction to Python dictionary comprehension
- Syntax for dictionary comprehension
- Modifying values
- Nested dictionary comprehension
- Advantages of dictionary comprehension
- Conclusion
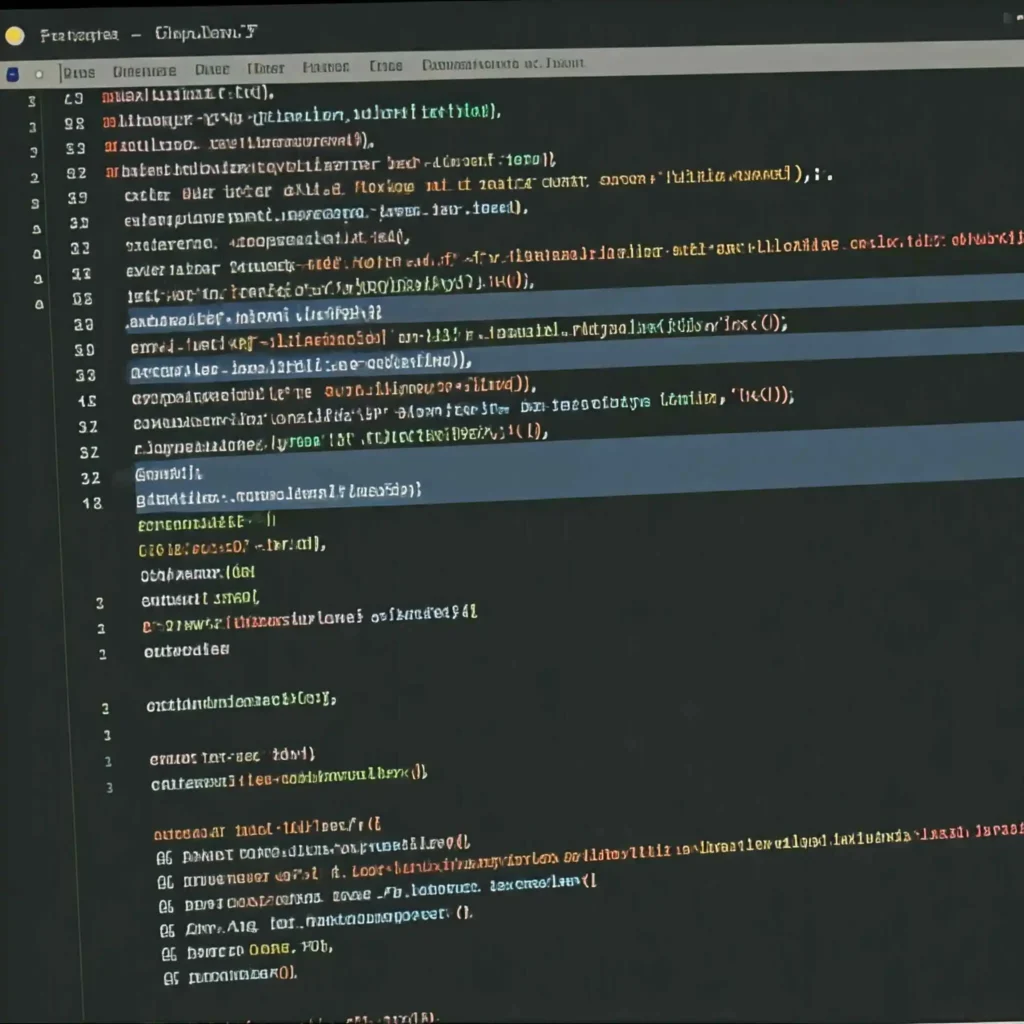
Introduction to Python dictionary comprehension
Python dictionary comprehension is a concise and an efficient way to create dictionaries. It allows you to construct a dictionary from an iterables. Such as a list, string or a tuple by just applying a condition on it.
It is very similar to the list comprehension but it is specifically for dictionaries. Which allows you to define key-value pairs in a single line of code.
Syntax for dictionary comprehension
{key_expression: value_expression for item in iterable if condition}
- key_expression: It defines the key of the dictionary.
- value_expression: It defines the value which will correspond to the key that we have defined.
- iterable: It is a collection which can be iterated like a list, a string or a tuple.
- condition (optional): It is a condition which we set that will help us filter the iterable and add them to the dictionary.
Example
# Creating a dictionary with numbers as keys and their squares as values
squares = {x: x**2 for x in range(5)}
print(squares)
Output
{0: 0, 1: 1, 2: 4, 3: 9, 4: 16}
In this example, for each x in the range 0-4, we calculate x**2 and use x as the key and use x**2 as the value.
Example with condition
We can filter items from the iterable using a condition which will only allow the items we want to add in the dictionary.
# Creating a dictionary with even numbers as keys and their squares as values
even_squares = {x: x**2 for x in range(10) if x % 2 == 0}
print(even_squares)
Output
{0: 0, 2: 4, 4: 16, 6: 36, 8: 64}
In this example, the condition adds only even numbers to the dictionary.
Modifying values
We can modify or manipulate values in the dictionary comprehension.
# Create a dictionary with numbers and their square roots
import math
square_roots = {x: math.sqrt(x) for x in range(1, 6)}
print(square_roots)
Output
{1: 1.0, 2: 1.4142135623730951, 3: 1.7320508075688772, 4: 2.0, 5: 2.23606797749979}
In this example, we have applied the square root function sqrt() which is from the math module to each number.
Nested dictionary comprehension
We can also create nested dictionary comprehension to create more complex dictionaries. It will do more tasks in less code.
# Creating a nested dictionary (a dictionary of dictionaries)
nested_dict = {x: {y: y**2 for y in range(3)} for x in range(3)}
print(nested_dict)
Output
{0: {0: 0, 1: 1, 2: 4}, 1: {0: 0, 1: 1, 2: 4}, 2: {0: 0, 1: 1, 2: 4}}
In the above example, we have created a dictionary where each key has a dictionary as a value. The corresponding dictionary has a key from 0 to 2 and value as square of the key.
Advantages of dictionary comprehension
- It is consice and reliable: It allows us to create dictionaries in a single line of code which makes it more readable.
- It is efficient: Dictionary comprehension is often faster than manually creating dictionaries using loops.
- It is flexible: We can apply condition and expressions directly.
Conclusion
Dictionary comprehension is a powerful tool which allows us to create dictionaries in clear, concise, efficient manner.
By understanding its syntax and knowing to applying conditions and expression, we can significantly reduce our code while maintaining and improving readability.
Leave a Reply