Table of Contents
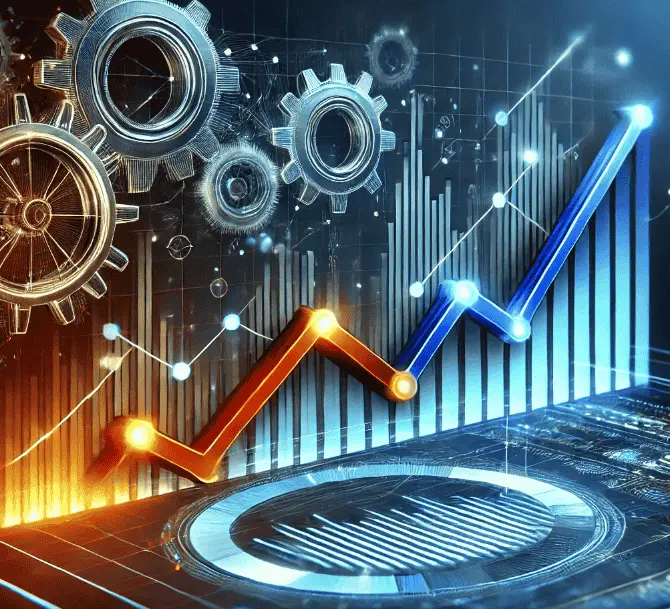
Introduction to Linear Regression
What is a linear regression?, Linear Regression is a statistical method to calculate the relationship between a two or more variables. The variables comes in two types, one is dependent variable and another is independent variable.
In linear regression, we use independent variables to derive dependent variables. We will see examples of these soon.
Why Python?, Python code is easy to understand and create. Moreover, we can directly import machine learning libraries to implement in our code.
What will you get from here?, We have created a complete begginner guide for Linear Regression in Python. You will know how linear regression works?, how can you use it?, from easily understandable examples.
Example: Evaluating model on sales data
In this example, we will evaluate linear regression on our sales data.
You can download sales data from here.
Installing necessary libraries
pip install pandas
pip install numpy
pip install scikit-learn
Example program: 1
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
import numpy as np
df = pd.read_csv('sales.csv')
X = df[['Price']]
y = df['Sales']
print("df")
print(df)
print("X")
print(X)
print("y")
print(y)
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=False)
# Initialize and train the Linear Regression model
model = LinearRegression()
model.fit(X_train, y_train)
predictions = model.predict(X)
df['Predictions'] = predictions
print(df)
Output of the above >>
Name: Sales, dtype: int64 Price Sales Predictions 0 500 50 58.776488 1 490 55 60.561951 2 460 65 65.918342 3 435 78 70.382001 4 462 65 65.561249 5 400 48 76.631123 6 430 73 71.274732 7 436 76 70.203454 8 425 78 72.167464 9 455 68 66.811074 10 432 73 70.917640 11 466 66 64.847064 12 465 65 65.025610 13 429 79 71.453279 14 459 61 66.096888 15 455 70 66.811074 16 449 79 67.882352 17 458 69 66.275434 18 441 74 69.310723 19 436 79 70.203454 20 460 60 65.918342 21 461 60 65.739795 22 440 78 69.489269 23 461 65 65.739795 24 422 80 72.703103 25 459 69 66.096888 26 458 63 66.275434 27 459 67 66.096888 28 460 65 65.918342 29 459 61 66.096888
How Linear Regression works
Linear Regression models the relationship between the independent variable (X) and the dependent variable (Y).
Formula: Y = mX + b
where, m is the slope of line i.e., how much Y changes for a unit change in X and b is value of Y when X = 0
Applications of Linear Regression
We can use it to predict future sales, stock trends or house prices. Moreover, one can make use of it to understand the relationship between two or more variables.
Also, we can use it to make baseline machine learning models.
Some things to consider
Before you start using Linear Regression in your projects, please take notice of these things.
Linear Regression assumes that the relationship between the variables is linear. As a result, the non-linear relationships can not be expressed as accurately as linear relationships.
Leave a Reply