Table of Contents
- Introduction to Python Exercises with Solutions
- Write a simple program that accomplises the following tasks
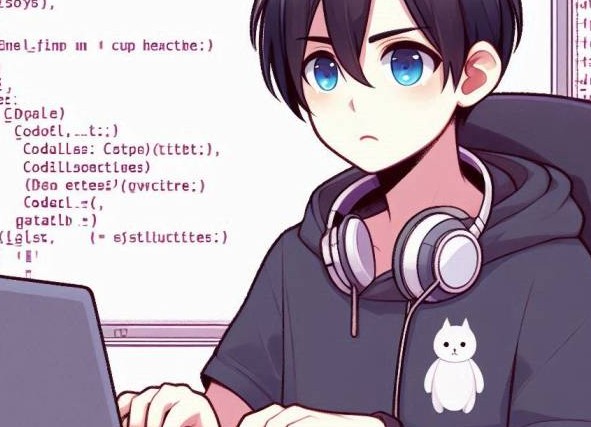
Introduction to Python Exercises with Solutions
You might be well-versed in Python programming, however, your click here suggests an interest in solving Python exercises. We have created Python exercises with solutions which are easy and helpful in understanding the basic concepts.
Write a simple program that accomplises the following tasks
1 Store your friends names in a list and then print each friends name each at a time.
Solution
my_friends = ['Jake','Amy','Calsie']
print(my_friends[0])
print(my_friends[1])
print(my_friends[2])
>>Jake
>>Amy
>>Calsie
2 Begin with the exercise(1) and print a personalised message to each of your friend.
Solution
my_friends = ['Jake','Amy','Calsie']
print("Hello " + my_friends[0] + " ,how are you?")
print("Hello " + my_friends[1] + " ,how are you?")
print("Hello " + my_friends[2] + " ,how are you?")
>>Hello Jake, how are you?
>>Hello Amy, how are you?
>>Hello Calsie, how are you?
3 Make your own list about tourist places, vehicles you like and print a message.
Solution
vehicles = ['BMW','Mercedes','Toyota','Bugati']
print("I would like to own a " + vehicles[0] + " one day.")
print("I would like to own a " + vehicles[1] + " one day.")
print("I would like to own a " + vehicles[2] + " one day.")
print("I would like to own a " + vehicles[3] + " one day.")
>>I would like to own a BMW one day.
>>I would like to own a Mercedes one day.
>>I would like to own a Toyota one day.
>>I would like to own a Bugati one day.
4 If you could invite anyone you wish, who would you invite?, make a list of persons who you would like to invite?, make a list of persons who you would like to invite to dinner and print a message to each person to invite them.
Solution
dinner_invite = ['Jake','Amy','Calsie','Ves','Benjamin']
print("Hello " + dinner_invite[0] + ", I would like to invite you to the dinner tonight, please come!")
print("Hello " + dinner_invite[1] + ", I would like to invite you to the dinner tonight, please come!")
print("Hello " + dinner_invite[2] + ", I would like to invite you to the dinner tonight, please come!")
print("Hello " + dinner_invite[3] + ", I would like to invite you to the dinner tonight, please come!")
print("Hello " + dinner_invite[4] + ", I would like to invite you to the dinner tonight, please come!")
>>Hello Jake, I would like to invite you to the dinner tonight, please come!
>>Hello Amy, I would like to invite you to the dinner tonight, please come!
>>Hello Calsie, I would like to invite you to the dinner tonight, please come!
>>Hello Ves, I would like to invite you to the dinner tonight, please come!
>>Hello Benjamin, I would like to invite you to the dinner tonight, please come!
5 You just came to know that one of your guest can’t make to the dinner, so you need to send out a new set of invitation. You’ll have to think of someone else to invite.
Solution
dinner_invite = ['Jake','Amy','Calsie','Ves','Benjamin']
cant_make = dinner_invite.pop()
print('Regrettably, ' + cant_make + ' can't make to the dinner due to some reasons, I will send a new invitations.')
print("Hello " + dinner_invite[0] + ", I would like to invite you to the dinner tonight, please come!")
print("Hello " + dinner_invite[1] + ", I would like to invite you to the dinner tonight, please come!")
print("Hello " + dinner_invite[2] + ", I would like to invite you to the dinner tonight, please come!")
print("Hello " + dinner_invite[3] + ", I would like to invite you to the dinner tonight, please come!")
>>Regrettably, Benjamin can't make to the dinner due to some reasons, I will send a new invitations.
>>Hello Jake, I would like to invite you to the dinner tonight, please come!
>>Hello Amy, I would like to invite you to the dinner tonight, please come!
>>Hello Calsie, I would like to invite you to the dinner tonight, please come!
>>Hello Ves, I would like to invite you to the dinner tonight, please come!
6 You just found a bigger dinner table, so you want to invite three more people now. Modify the list to add three more people at a start, at the middle and at last.
Solution
dinner_invite = ['Jake','Amy','Calsie','Ves','Benjamin']
print('I recently found a bigger table, I can invite more people to the dinner!!')
dinner_invite.insert(0, 'Mark')
dinner_invite.insert(3, 'Quin')
dinner_invite.append('Henry')
print("Hello " + dinner_invite[0] + ", I would like to invite you to the dinner tonight, please come!")
print("Hello " + dinner_invite[1] + ", I would like to invite you to the dinner tonight, please come!")
print("Hello " + dinner_invite[2] + ", I would like to invite you to the dinner tonight, please come!")
print("Hello " + dinner_invite[3] + ", I would like to invite you to the dinner tonight, please come!")
print("Hello " + dinner_invite[4] + ", I would like to invite you to the dinner tonight, please come!")
print("Hello " + dinner_invite[5] + ", I would like to invite you to the dinner tonight, please come!")
print("Hello " + dinner_invite[6] + ", I would like to invite you to the dinner tonight, please come!")
print("Hello " + dinner_invite[7] + ", I would like to invite you to the dinner tonight, please come!")
>>I recently found a bigger table, I can invite more people to the dinner!!
>>Hello Mark, I would like to invite you to the dinner tonight, please come!
>>Hello Jake, I would like to invite you to the dinner tonight, please come!
>>Hello Amy, I would like to invite you to the dinner tonight, please come!
>>Hello Calsie, I would like to invite you to the dinner tonight, please come!
>>Hello Quin, I would like to invite you to the dinner tonight, please come!
>>Hello Ves, I would like to invite you to the dinner tonight, please come!
>>Hello Benjamin, I would like to invite you to the dinner tonight, please come!
>>Hello Henry, I would like to invite you to the dinner tonight, please come!
7 Think of at least 5 places in the world you would like to visit.
(i) Make a list of these places, make sure it is not in alphabetical order.
(ii) Print your list in orignal order.
(iii) Use sorted() function to print the list in alphabetical order.
(iv) Print the orignal list showing that is isn’t modified.
Solution
visit_places = ['Petra','Colosseum','Machu Picchu','Tokyo','New York']
print("Printing orignal list: ")
print(visit_places)
print("Printing sorted list: ")
print(sorted(visit_places))
print("Printing the orignal list again: ")
print(visit_places)
>>Printing orignal list:
>>['Petra','Colosseum','Machu Picchu','Tokyo','New York']
>>Printing sorted list:
>>['Colosseum','Machu Picchu','New York','Petra','Tokyo']
>>Printing the orignal list again:
>>['Petra','Colosseum','Machu Picchu','Tokyo','New York']
8 Intentional Error: If you haven’t received an index error, try to make one happen.
Solution
visit_places = ['Petra','Colosseum','Machu Picchu','Tokyo','New York']
print(visit_places[0]
print(visit_places[2])
print(visit_places[5])
>>Petra
>>Machu Picchu
>>Traceback (most recent call last):
>>File "", line 1, in
>>IndexError: list index out of range
9 Think of at least three kinds of your favourite pizzas, store these pizza names in a list, and then use a for loop to print each pizza.
Solution
av_pizzas = ['Cheese Pizza','Mushroom Pizza','Veggies Pizza']
for name in fav_pizzas:
print(name)
>>Cheese Pizza
>>Mushroom Pizza
>>Veggies Pizza
10 Think of at least three different animals that are domestic. Use a for loop to print the animal name along with a message.
Solution
domestic_animals = ['Cat','Dog','Rabbit','Parrot','Hamster','Lemming','Goose']
for animal in domestic_animals:
print("The " + animal + " is a domestic animal.")
>>The Cat is a domestic animal.
>>The Dog is a domestic animal.
>>The Rabbit is a domestic animal.
>>The Parrot is a domestic animal.
>>The Hamster is a domestic animal.
>>The Lemming is a domestic animal.
>>The Goose is a domestic animal.
Leave a Reply