Table of Contents
- Introduction to Fyers API Stock Data
- Getting data by duration
- Benefits of using Fyers API stock Data
- Frequent Asked Questions
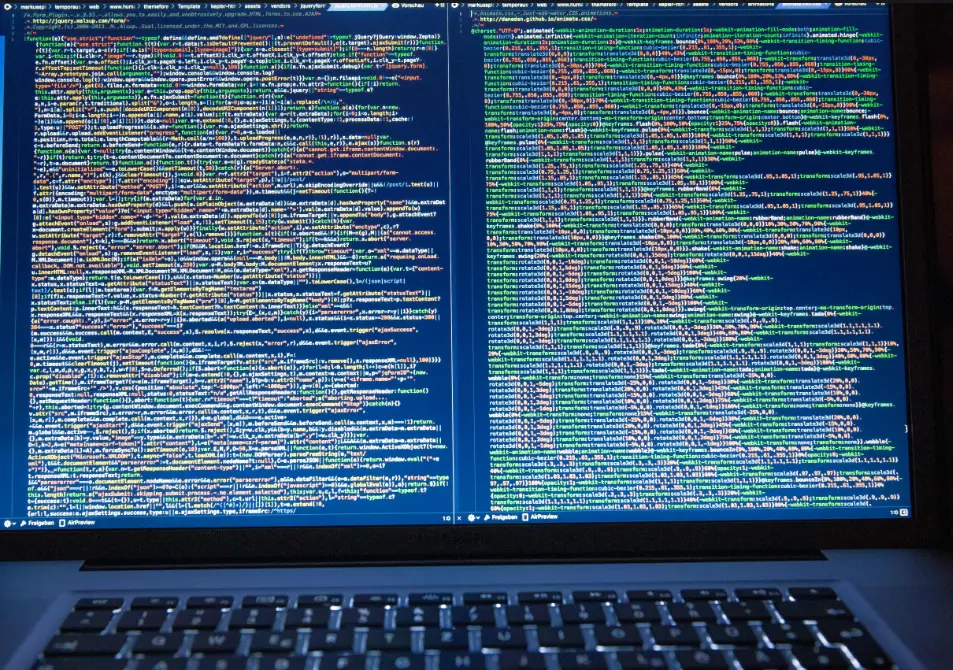
Introduction to Fyers API Stock Data
In our previous posts, we have seen how can we login and get our status and profile data, we have also seen that how can we get live Data from the symbols. In this post, we will get stock Data using Fyers API stock Data by duration and date.
Getting data by duration
We will explore a program that allows us to fetch data for a specific symbol and duration of our choice.
The duration can be 1 day to 90 days and the resolution can be 5s to 240m. View the complete list from here.
Let us start with an example program.
#Fetches data and returns a dataframe for symbol
#Input - symbol_name, duration - start and end time in date, resolution
from fyers_apiv3 import fyersModel
import pandas as pd
import datetime as dt
with open('access.txt', 'r') as a:
access_token = a.read()
client_id = 'your client id'
# Initialize the FyersModel instance with your client_id, access_token, and enable async mode
fyers = fyersModel.FyersModel(client_id=client_id, is_async=False, token=access_token, log_path="")
class FetchData():
"""Fetches data and returns it in dataframe form"""
def __init__(self):
pass
def fetchByDate(self,symbol,resolution, duration):
"""Fetches data by Date"""
# Duration
duration = int(duration)
today_d = dt.date.today()
before_d = dt.date.today() - dt.timedelta(duration)
data = {
"symbol": symbol,
"resolution": str(resolution),
"date_format": "1",
"range_from": before_d,
"range_to": today_d,
"cont_flag": "1"
}
sdata2 = fyers.history(data=data)
sdata1 = sdata2['candles']
sdata = pd.DataFrame(sdata1)
sdata.columns = ['date', 'Open', 'High', 'Low', 'Close', 'Volume']
sdata['date'] = pd.to_datetime(sdata['date'], unit='s')
sdata.date = (sdata.date.dt.tz_localize('UTC').dt.tz_convert('Asia/Kolkata'))
sdata['date'] = sdata['date'].dt.tz_localize(None)
# Convert 'DateTime' column to datetime dtype
sdata['date'] = pd.to_datetime(sdata['date'])
#return sdata
return sdata
var1 = FetchData()
var1 = var1.fetchByDate('NSE:TECHM-EQ','1','2')
print(var1)
This is the complete code that you can use to retrieve data based on duration. You just need to input the required values to get the data. Now, let’s break down the code to better understand how it works.
Disassembling the code
We are importing fyers, pandas and datetime library. These only we need this time since we are not going to use live data.
#Fetches data and returns a dataframe for symbol
#Input - symbol_name, duration - start and end time in date, resolution
from fyers_apiv3 import fyersModel
import pandas as pd
import datetime as dt
Logging in
As mentioned earlier, we need to log in to the API and retrieve the access token for use. The first three lines open the text file where the access token is stored and assign it to the access_token
variable.
The next line initializes the Fyers model, which will be used whenever we call ‘fyers’.
with open('access.txt', 'r') as a:
access_token = a.read()
client_id = 'your client id'
# Initialize the FyersModel instance with your client_id, access_token, and enable async mode
fyers = fyersModel.FyersModel(client_id=client_id, is_async=False, token=access_token, log_path="")
Making a Class
We will create a class FetchData(), it will contain more than one function that we are going to define.
class FetchData():
"""Fetches data and returns it in dataframe form"""
Defining functions
As mentioned earlier, we need to log in to the API and obtain the access token for use. The first three lines open the text file containing the access token and assign it to the access_token
variable.
The following line initializes the Fyers model, which we will use whenever we reference ‘fyers’.
def __init__(self):
pass
def fetchByDate(self,symbol,resolution, duration):
"""Fetches data by Date"""
Converting duration to start and end date
This code takes the duration provided to the function and converts it into a start and end date.
For example, if we provide a duration of 10 days, the start date will be 10 days before today, and the end date will be today.
# Duration
duration = int(duration)
today_d = dt.date.today()
before_d = dt.date.today() - dt.timedelta(duration)
Making a dictionary of all the information
It is a dictionary that will be populated with the symbol name, start date, end date, and resolution. All this data will be added to the dictionary in the following way.
data = {
"symbol": symbol,
"resolution": str(resolution),
"date_format": "1",
"range_from": before_d,
"range_to": today_d,
"cont_flag": "1"
}
Providing data to the API
The data dictionary will be sent to the Fyers API in this manner. The API will return the data, which will then be stored in the sdata2
variable.
We have obtained the data, but it requires some modifications. Since the data is in dictionary format and the time is in epoch format, we will adjust it to meet our needs.
sdata2 = fyers.history(data=data)
Modifying the received data to get the date column correctly
First, we extract the ‘candle’ dictionary from the API response and assign it to the sdata1 variable.
Subsequently, we create a pandas DataFrame using sdata1 and store it in the sdata variable. Finally, we rename the columns of the DataFrame to ‘date’, ‘open’, ‘high’, ‘low’, ‘close’, and ‘volume’.
Afterwards, we convert the date format to Indian Standard Time.
The final line returns the sdata
, which is the processed data the function has worked on.
sdata1 = sdata2['candles']
sdata = pd.DataFrame(sdata1)
sdata.columns = ['date', 'Open', 'High', 'Low', 'Close', 'Volume']
sdata['date'] = pd.to_datetime(sdata['date'], unit='s')
sdata.date = (sdata.date.dt.tz_localize('UTC').dt.tz_convert('Asia/Kolkata'))
sdata['date'] = sdata['date'].dt.tz_localize(None)
# Convert 'DateTime' column to datetime dtype
sdata['date'] = pd.to_datetime(sdata['date'])
#return sdata
return sdata
Benefits of using Fyers API stock Data
When we trade with using our mobile phones, we can view limited amount of data at any specific instant. The small screens makes it easy to ignore smaller important details while we were Analysing Data of about a month or two.
But this problem does not exist if you successfully make a program which can help you gaining insights to the collected data.
We will update our posts regularly and will continue to add quality content. If you have any requests regarding the program, you can comment us.
Frequent Asked Questions
Yes and No, you can copy it and run it in your Python terminal but you have to add your information in the code such as client-ID and access token.
Also, you can modify symbol name and date as per your requirements.
You can comment us if you have any problem, we will be there to assist you.
Leave a Reply