Table of Contents
- Introduction to Facebook Prophet
- Why you should use Facebook Prophet
- Preparing environment for Forecasting
- Forcasting using Facebook Prophet
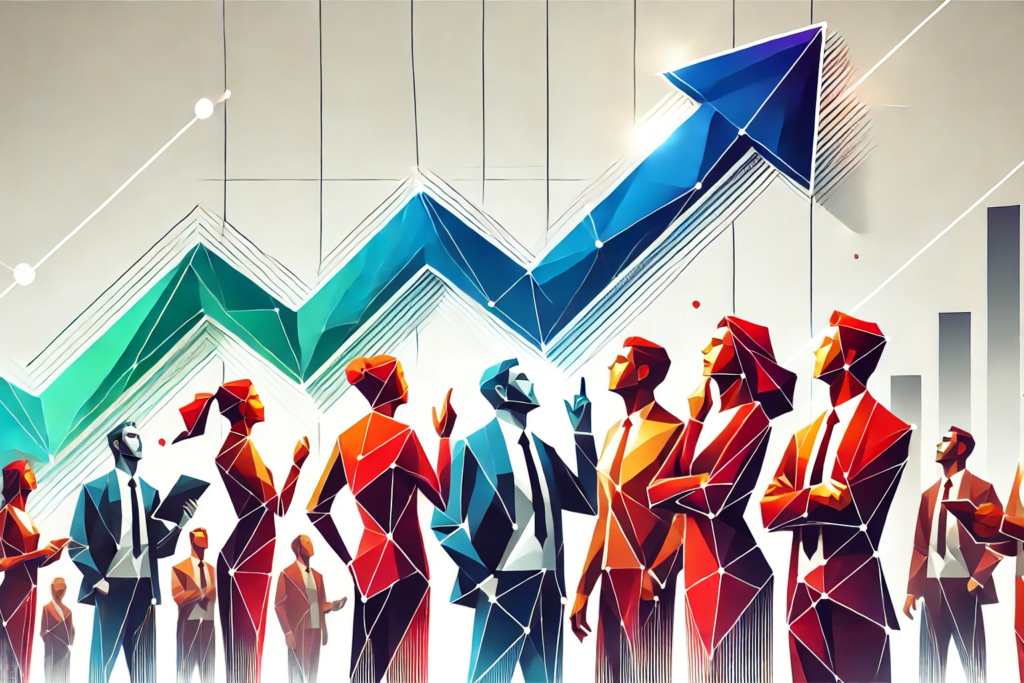
Introduction to Facebook Prophet
Facebook Prophet is an open-source forecasting tool developed by Facebook. It can make accurate forecasts on time-series data. Even when dealing with seasonality, holidays and other patterns which are common in real world.
This is particularly useful for business forecasting such as predicting sales or stock demand.
How It works
It uses an additive model that combines the trend of data. By doing this, it achieves the overall upward and downward trend of the data over time.
It models periodic patterns like yearly, monthly and weekly fluctuation while also accounting for existing holidays in the data.
Why you should use Facebook Prophet
Facebook Prophet is popular, because its user-friendly and requires minimal configuration, while producing reliable results for business applications.
Unlike other time-series models, it can work well with messy data as well as irregular sampling which is common in the real world.
Preparing environment for Forecasting
Installation of Facebook Prophet
We can easily install Facebook Prophet by writing this command in the terminal
pip install prophet
Importing libraries
To start forecasting, we need to import the its library into our project.
import pandas as pd
from prophet import Prophet
Data to Forecast
Now our environment is ready, we need the data to forecast. We will start from forecasting the below data.
Forcasting using Facebook Prophet
Example 1: Forecasting Electric production
We have electricity production data in csv 1, we will predict the future electricity production using this data.
In the below code, we have imported Prophet and Pandas library. After that, we have stored csv data into a dataframe named df. We have them printed its last 5 rows.
import pandas as pd
from prophet import Prophet
#reading and storing csv data in a dataframe
df = pd.read_csv('https://paradisecoder.com/wp-content/uploads/2024/12/Electric_Production-Sheet1.csv')
#printing dataframe last 5 values
print(df.tail())
Output of the above >>
ds y 392 9/1/2017 98.6154 393 10/1/2017 93.6137 394 11/1/2017 97.3359 395 12/1/2017 114.7212 396 1/1/2018 129.4048
We have called Prophet model in variable m and then fittet our data in it which is a dataframe df.
#calling model
m = Prophet()
#fitting our data into the model
m.fit(df)
After that, we have made 10 additional data points in the future and stored it in future variable and printed its last 5 values using print function.
#making 10 data points in future for predicting
future = m.make_future_dataframe(periods=10)
#printing 5 values
print(future.tail())
Output of the above >>
ds 402 2018-01-07 403 2018-01-08 404 2018-01-09 405 2018-01-10 406 2018-01-11
Now, we have used this model to predict 10 values and store it in forecast variable.
Afterwards, we have printed last 5 values of the selected columns.
#forecasting data using model
forecast = m.predict(future)
#naming columns and printing it
print(forecast[['ds', 'yhat', 'yhat_lower', 'yhat_upper']].tail())
Output of the above >>
ds yhat yhat_lower yhat_upper 402 2018-01-07 119.983528 116.293831 123.725298 403 2018-01-08 121.357068 117.630464 124.935681 404 2018-01-09 122.864152 119.274042 126.459013 405 2018-01-10 124.450675 121.039400 128.114974 406 2018-01-11 126.060184 122.775125 129.886945
Leave a Reply