Table of Contents
- Key Points about Python unit test:
- Example of a Simple Unit Test in Python:
- CommRunning Unit Tests:
- Running Unit Tests:
- Unit Testing Best Practices:
- Conclusion of Python unit test:
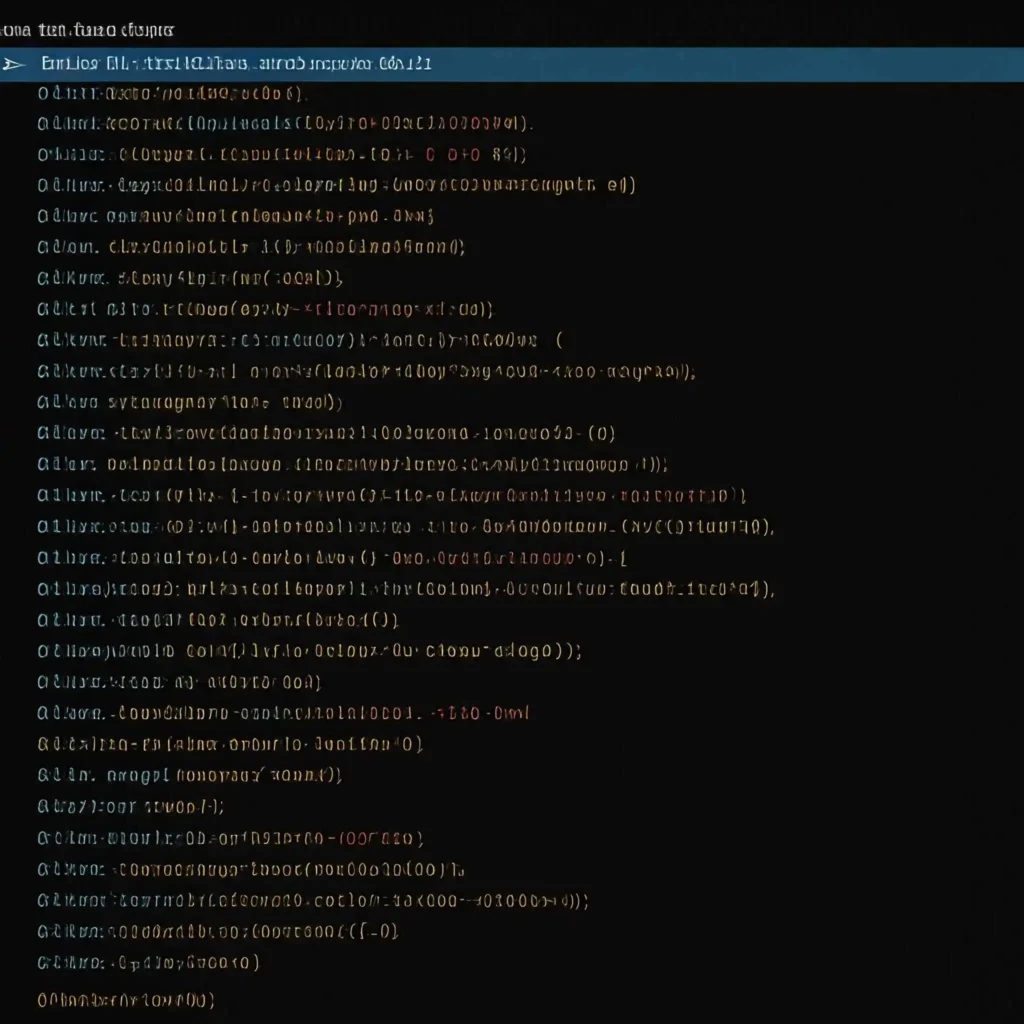
We can test the correctness of a small, individual units of code. Such as functions of code with the help of Python unit test.
We can check if a functionality in a program works as expected. When we isolate it from the rest of the program.
This helps us identify the bugs in the development phase and also determine the logic is working as we expect.
Key Points about Python unit test:
- Purpose:
- The primary purpose of unit tests is to test a single “unit” of code (e.g., a function or a method) in isolation, ensuring that it behaves correctly for a range of inputs.
- Unit tests provide confidence that code changes do not break existing functionality.
- Unit Test Framework in Python:
- Python provides a built-in module called
unittest
for writing and running unit tests. This module includes tools for defining test cases, running tests, and generating test reports.
- Python provides a built-in module called
- Structure:
- A unit test typically includes:
- Test Case: A method that checks a specific piece of functionality.
- Assertions: Statements that check if the output of a function matches the expected result.
- Test Runner: A mechanism to run the tests and report results.
- A unit test typically includes:
- Advantages of Unit Testing:
- Early Bug Detection: Unit tests help find bugs at the unit level before the code is integrated with other parts of the application.
- Improved Code Quality: Writing unit tests encourages cleaner, modular code.
- Refactoring Safety: Unit tests help ensure that refactoring or code changes do not introduce new bugs.
- Documentation: Unit tests serve as documentation for the expected behavior of functions.
Example of a Simple Unit Test in Python:
import unittest
# Function to be tested
def add(a, b):
return a + b
# Unit test class
class TestMathOperations(unittest.TestCase):
def test_add(self):
# Test for the add function
result = add(2, 3)
self.assertEqual(result, 5) # Assert that the result is 5
def test_add_negative(self):
# Test with negative numbers
result = add(-2, 3)
self.assertEqual(result, 1) # Assert that the result is 1
def test_add_zero(self):
# Test with zero
result = add(0, 0)
self.assertEqual(result, 0) # Assert that the result is 0
# Running the tests
if __name__ == '__main__':
unittest.main()
Key Components in the Example:
- Function Under Test:
add(a, b)
is the function being tested. - Test Class:
TestMathOperations
is a subclass ofunittest.TestCase
. Each method inside this class is a test case. - Test Methods: Methods like
test_add()
,test_add_negative()
, andtest_add_zero()
test different aspects of theadd()
function. - Assertions:
self.assertEqual(result, 5)
checks if the result of the function call matches the expected value. - Test Runner:
unittest.main()
runs the tests when the script is executed.
CommRunning Unit Tests:
self.assertEqual(a, b)
: Check ifa == b
.self.assertNotEqual(a, b)
: Check ifa != b
.self.assertTrue(x)
: Check ifx
isTrue
.self.assertFalse(x)
: Check ifx
isFalse
.self.assertIsNone(x)
: Check ifx
isNone
.self.assertIsInstance(x, type)
: Check ifx
is an instance of the given type.
Running Unit Tests:
To run unit tests, you can simply execute the script or use a test runner. When using the unittest
module, you can run tests from the command line:
Bash
python -m unittest test_module.py
Or run the tests directly if using a script like the one above.
Unit Testing Best Practices:
- Write Tests for Edge Cases: Test with both normal and edge cases to ensure comprehensive coverage.
- Keep Tests Isolated: Each unit test should focus on a single function or unit of code. Avoid testing multiple things in one test.
- Use Mocking: When testing units that depend on external services (e.g., databases, APIs), use mocking to isolate the unit being tested.
- Test Every Function: Aim to write unit tests for every function or method in your codebase to ensure maximum test coverage.
Conclusion of Python unit test:
Unit tests are essential for verifying that individual pieces of code work as expected.
They help ensure that code changes don’t introduce regressions, improve code quality, and act as a form of documentation.
Using Python’s unittest
module, you can write and execute unit tests to make sure your functions behave correctly across various scenarios.
Leave a Reply