Table of Contents
- Introduction to Python’s paramter passing system
- Types of Arguments in Python’s paramter passing system:
- Conclusion:
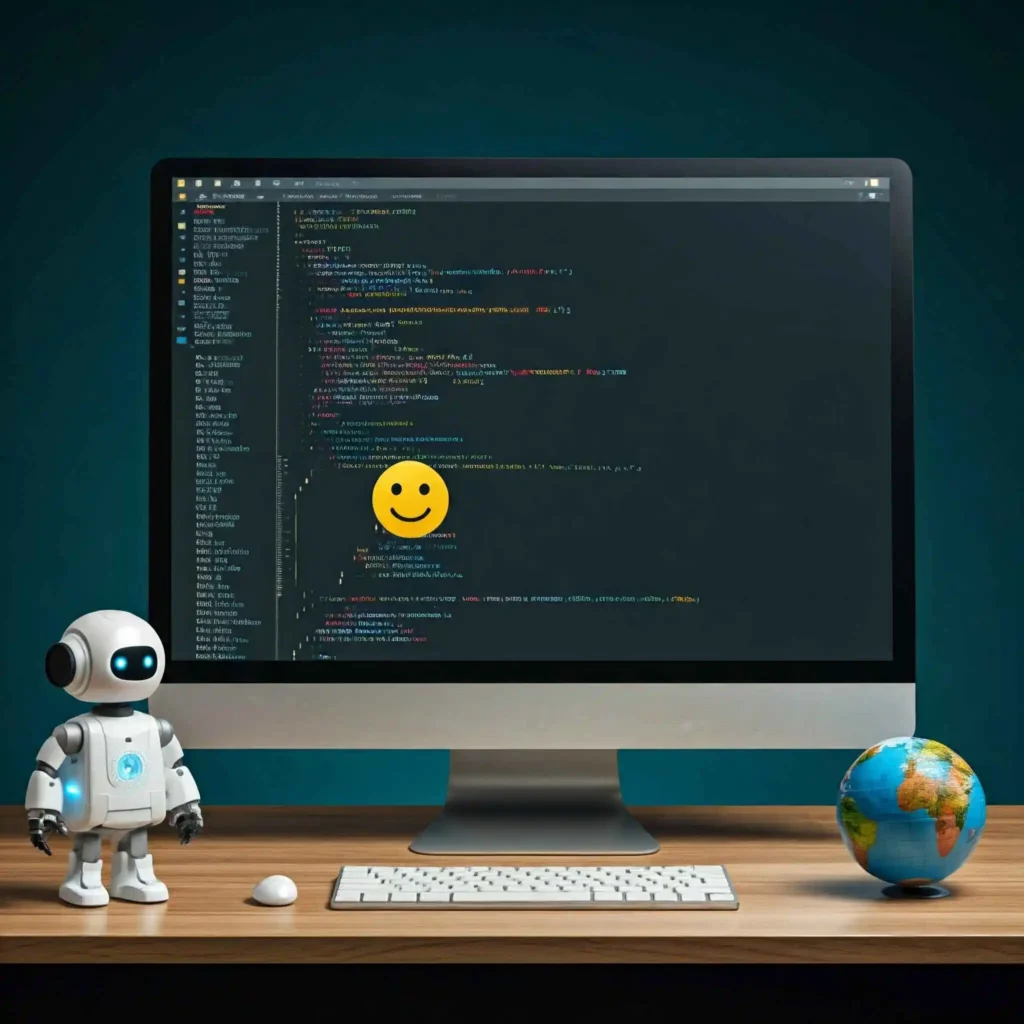
Introduction to Python’s paramter passing system
The Python’s parameter passing system is often a topic of confusion, but understanding how it works is important.
Python uses a mechanism known as “pass-by-object-reference” (sometimes called “pass-by-assignment”). Here’s a detailed explanation of how it works:
Pass-by-Object-Reference:
In Python, variables hold references to objects in memory, rather than the actual data. So, when you pass a variable to a function, you are passing a reference to the object that the variable points to, not the actual object itself.
Python’s parameter passing system is sometimes referred to as “pass-by-object-reference”. It means that when you pass an argument to a function, you are passing a reference to the object, but not the reference itself.
This allows functions to modify mutable objects (like lists, dictionaries, etc.), but not immutable objects (like integers, strings, and tuples).
Types of Arguments in Python’s paramter passing system:
Python treats parameters as references to objects, and how they behave depends on whether the objects are mutable or immutable:
1. Mutable Objects (e.g., lists, dictionaries, sets):
When you pass a mutable object to a function, the function can modify the object in place.
def modify_list(my_list):
my_list.append(10) # Modifies the original list
lst = [1, 2, 3]
modify_list(lst)
print(lst) # Output: [1, 2, 3, 10] (list is modified)
In this case, since lst
is mutable (a list), any changes made to it inside the function will affect the original object.
2. Immutable Objects (e.g., integers, strings, tuples):
When you pass an immutable object to a function, the object itself cannot be changed. If the function attempts to modify the object, a new object is created, and the reference inside the function will point to the new object, not affecting the original object.
def modify_number(x):
x = 5 # Reassigning, creates a new integer object
num = 10
modify_number(num)
print(num) # Output: 10 (original integer is unchanged)
In this case, since num
is immutable (an integer), the original value remains unchanged outside the function.
Key Points:
- Function Argument Behavior:
- If the argument is mutable, the function can modify the object.
- If the argument is immutable, the function cannot change the object’s value, but it can rebind the reference to a new object.
- What Python Passes:
- Python passes references to objects, not the actual objects themselves.
- This means you can alter mutable objects within the function, but immutable objects will appear unchanged unless the function rebinds the reference to a new object.
- Assignment vs. Mutation:
- Mutation: When the object is modified in place (e.g., modifying a list).
- Rebinding: When the variable reference is changed to point to a new object (e.g., assigning a new value to an integer or string).
Conclusion:
In Python, the parameter passing system is best described as pass-by-object-reference. It means that functions receive references to the objects that are passed in. The behavior of the object depends on whether it’s mutable or immutable:
- For mutable objects, the function can modify the object.
- For immutable objects, the function cannot change the object itself but can rebind the reference to a new object.
Leave a Reply