Table of Contents
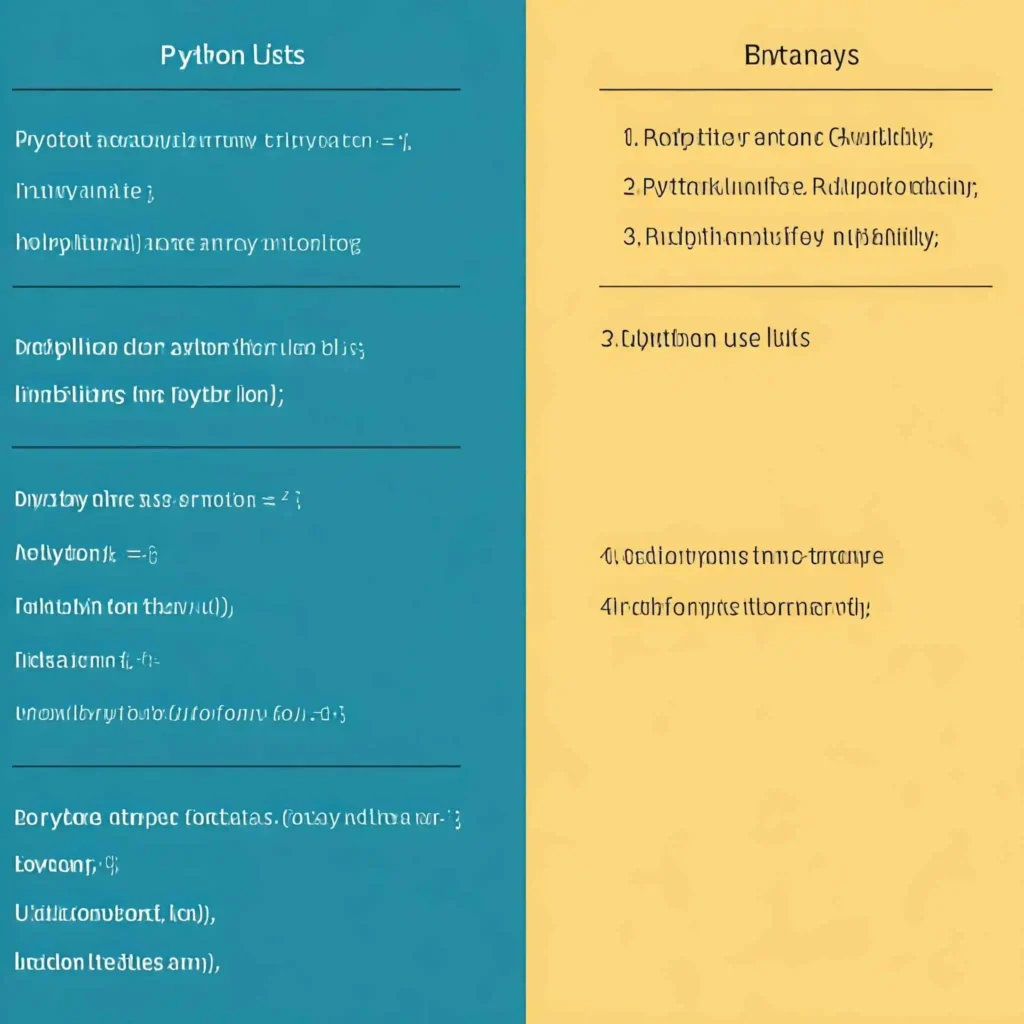
We use both the array and the list to store data but there are difference between Python array and Python list.
1. Definition and Data Structure:
Python list
A list is a built-in data structure in Python that can store a collection of elements.Lists can hold elements of different data types (e.g., integers, strings, floats, etc.) in the same list.
Lists are implemented as dynamic arrays in Python, which means they can grow and shrink in size dynamically.Lists are very flexible and can be used for a variety of tasks.
For Example:
my_list = [1, "apple", 3.14, True]
Python Array
An array is a specialized data structure provided by the array
module.
Arrays can only store elements of the same data type. The type of elements (e.g., integers, floats) is specified when the array is created.
Arrays are more memory efficient than lists because they store elements in a contiguous block of memory and don’t require overhead for storing different types.
import array
my_array = array.array('i', [1, 2, 3, 4]) # 'i' indicates an array of integers
Homogeneity:
Python List
Can store elements of multiple data types in a single list.
mixed_list = [1, "apple", 3.14]
Python Array
Can store elements of only one data type. You need to define the type of elements (like integers, floats, etc.) when creating the array.
import array
int_array = array.array('i', [1, 2, 3, 4]) # Only integers are allowed
Flexibility:
Python List
Lists are more flexible and can store different types of elements. You can append, remove, or modify elements easily without worrying about the data type.
Python Array
Arrays are less flexible because they are intended for homogenous data. Once created with a specific type, they cannot store other types of data without conversion.
Performance:
Python List
Lists can be slower for large collections of numeric data because they store references to objects and require more memory. Lists use more memory due to their flexibility in storing mixed data types.
Python Array
Arrays are more memory-efficient for large numeric data. They are typically faster for numerical computations because they are tightly packed in memory and do not need to store type information for each element individually.
Use Case:
Python List
Suitable for most general-purpose programming tasks. Lists are the default choice for storing a collection of items, especially when the elements can vary in type.
Array
Best suited for situations where you are dealing with large collections of data of a single type, and performance or memory efficiency is important, especially for numerical operations or data manipulation (e.g., when working with large sets of numbers).
Functionality:
Python List
List come with a wide variety of built-in methods, e.g., append(), extend(), pop(), remove(), insert(), sort(), reverse(), making them more versatile.
Python Array
Arrays are more limited in terms of methods compared to lists. The array
module provides methods like append()
, remove()
, pop()
, and insert()
, but they are primarily designed for managing homogenous data types efficiently.
Size:
Python List
Lists are dynamic in size. They can grow and shrink automatically as elements are added or removed.
Python Array
Arrays also grow and shrink automatically, but their resizing behavior is optimized for performance when dealing with large data sets of the same type.
Syntax for Declaration:
Python List
my_list = [1, 2, 3, 4, "hello", 3.14] # Mixed data types allowed
Python Array
import array
my_array = array.array('i', [1, 2, 3, 4]) # 'i' is for integers
Example comparison:
# List Example
my_list = [1, "hello", 3.14, True]
my_list.append("world")
print(my_list) # Output: [1, 'hello', 3.14, True, 'world']
# Array Example
import array
my_array = array.array('i', [1, 2, 3, 4]) # 'i' specifies integer type
my_array.append(5)
print(my_array) # Output: array('i', [1, 2, 3, 4, 5])
Conclusion of differences between Python arrays and Python lists
- Use Python arrays when you are dealing with large amounts of homogeneous data (such as numbers) and need better memory efficiency or performance for numerical operations.
- Use Python lists when you need to store a collection of items of potentially different types, or when you need a more flexible data structure.
Leave a Reply